Forms are an essential part of any web application, and Angular provides us with powerful tools to create forms with validation without the need for any additional libraries. In this article, we will explore how to create forms in Angular using both reactive forms and template-driven forms, and how to retrieve form values from each type.
What are the Types of Angular Forms?
There are two types of forms in Angular – reactive forms and template-driven forms. Each has its own advantages and use cases, so it’s important to understand the differences between them before deciding which one to use in your project.
Reactive Forms
Reactive forms allow us to create forms by directly accessing the form values from the form object. This makes them more scalable and reusable compared to template-driven forms. The data flow in reactive forms is synchronous, and the form data model is immutable, meaning that once a value is set, it cannot be changed.
To use reactive forms in our Angular app, we need to import the ReactiveFormsModule in our app.module.ts file:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { ReactiveFormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
ReactiveFormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Once we have imported the ReactiveFormsModule, we can start creating our forms using the FormGroup and FormControl classes provided by Angular.
Template-Driven Forms
Template-driven forms, on the other hand, bind form values to variables that can be accessed using directives. This approach is more suitable for simple forms with less complex validation requirements. Unlike reactive forms, the data model in template-driven forms is mutable, meaning that values can be changed after they have been set.
To use template-driven forms, we need to import the FormsModule in our app.module.ts file:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
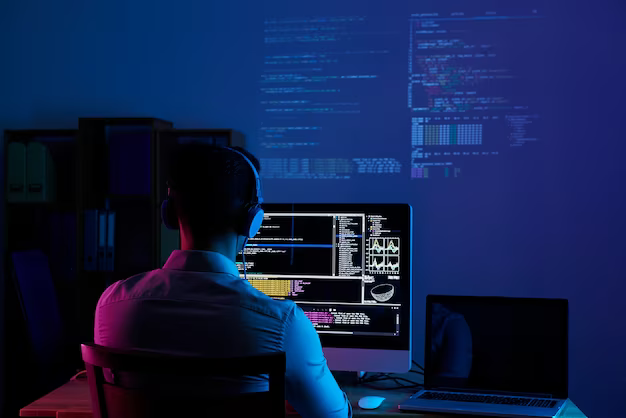
How Do You Get Form Values with Reactive Forms?
Now that we have a basic understanding of reactive forms, let’s dive into how we can retrieve form values using this approach.
Creating a Form
To create a form using reactive forms, we first need to define a FormGroup object in our component. This object represents the entire form and contains all the form controls and their values. We can then add individual form controls using the FormControl class.
Let’s say we want to create a simple login form with an email and password field. We can define our form in our component as follows:
import { Component } from '@angular/core';
import { FormGroup, FormControl } from '@angular/forms';
@Component({
selector: 'app-login',
templateUrl: './login.component.html',
styleUrls: ['./login.component.css']
})
export class LoginComponent {
loginForm = new FormGroup({
email: new FormControl(),
password: new FormControl()
});
}
In the above code, we have defined a FormGroup named loginForm, which contains two `FormControl`s – one for the email field and one for the password field.
Binding Form Controls to HTML Elements
Next, we need to bind our form controls to the corresponding HTML elements in our template. We can do this using the formControlName directive provided by Angular. Let’s update our login.component.html file to include our form:
<form [formGroup]="loginForm">
<label>Email:</label>
<input type="email" formControlName="email">
<label>Password:</label>
<input type="password" formControlName="password">
<button type="submit">Login</button>
</form>
In the above code, we have used the formControlName directive to bind our form controls to the email and password input fields.
Retrieving Form Values
Now that we have our form set up, we can retrieve the form values using the value property of the FormControl objects. We can access these values in our component class as follows:
onSubmit() {
console.log(this.loginForm.value);
}
The onSubmit() method will be called when the user clicks on the login button. It will log the form values to the console, which we can then use for further processing.
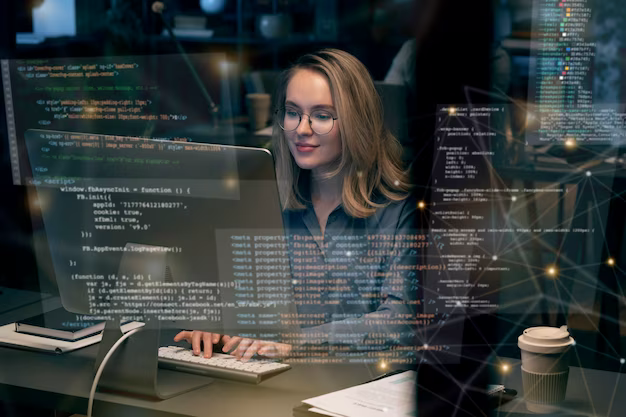
Are Template-Driven Forms Effective?
Template-driven forms follow a different approach compared to reactive forms. Instead of directly accessing the form values from the form object, we need to bind them to variables using directives. Let’s see how we can achieve this.
Creating a Form
To create a form using template-driven forms, we first need to add the ngModel directive to our form elements. This directive allows us to bind form values to variables in our component class. Let’s update our login.component.html file to use template-driven forms:
<form
# loginForm="ngForm">
<label>Email:</label>
<input type="email" [(ngModel)]="email" name="email">
<label>Password:</label>
<input type="password" [(ngModel)]="password" name="password">
<button type="submit">Login</button>
</form>
In the above code, we have added the ngModel directive to our input fields and bound them to variables named email and password.
Retrieving Form Values
To retrieve form values in template-driven forms, we can simply access the variables that are bound to our form controls. In our component class, we can define these variables as follows:
import { Component } from '@angular/core';
@Component({
selector: 'app-login',
templateUrl: './login.component.html',
styleUrls: ['./login.component.css']
})
export class LoginComponent {
email: string;
password: string;
onSubmit() {
console.log(this.email, this.password);
}
}
In the above code, we have defined two variables – email and password – which will hold the values entered by the user in the form. We can then use these variables for further processing, such as sending them to an API or performing client-side validation.
Request Filtering Denies File Extension
In the realm of web application security, request filtering plays a pivotal role in fortifying systems against potential vulnerabilities. One such measure involves denying access to files based on their extensions. By configuring request filtering rules, web developers can proactively thwart malicious attempts to exploit vulnerabilities associated with specific file types. Implementing robust request filtering mechanisms is imperative for bolstering the security posture of web applications and safeguarding sensitive data from unauthorized access.
Conclusion
In this article, we explored how to create forms in Angular using both reactive forms and template-driven forms, and how to retrieve form values from each type. We also discussed the differences between these two approaches and their use cases. By now, you should have a good understanding of how to create forms in Angular and how to get form values using different methods.