Building web applications with Angular can be a complex process, but there are ways to make it easier. One of these ways is by using pre-built components to create the frontend of our apps. And when it comes to user interface components, Bootstrap is one of the most popular choices. Bootstrap provides a wide range of UI components that allow us to create visually appealing and interactive frontends with ease.
Installing ng-bootstrap
The easiest way to add Bootstrap components into our Angular apps is by using the ng-bootstrap library. This library creates Angular components based on the vanilla version of Bootstrap, making it easy for us to add ready-to-use Bootstrap components into our Angular projects.
To install ng-bootstrap, we can use the following command:
ng add @ng-bootstrap/ng-bootstrap
This command utilizes the Angular CLI and can be used with most Angular projects. Once executed, it will automatically add all the necessary dependencies to our Angular modules, allowing us to use the provided components right away.
It’s worth noting that the latest version of ng-bootstrap is based on the latest version of Bootstrap. So, before proceeding with the installation, make sure to have the latest version of Bootstrap installed in your project.
After running the command, we should see something like this in our app.module.ts file:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
Adding Common Components
Now that we have ng-bootstrap installed, we can start adding some common Bootstrap components into our Angular app. In this section, we will go through some of the most commonly used components and see how we can integrate them into our project.
Alert
The Alert component in Bootstrap is used to display a short, important message to the user. It can be used for various purposes, such as displaying success or error messages. To add an Alert component in our Angular app, we can use the following code:
<ngb-alert [dismissible]="false" type="success">
This is a success alert!
</ngb-alert>
This will display a green-colored alert with the text “This is a success alert!” inside it. We can also make the alert dismissible by setting the dismissible attribute to true. Additionally, we can change the type of the alert by changing the type attribute to warning, danger, or info.
Date Picker
Date pickers are essential when working with forms that require users to input dates. With ng-bootstrap, we can easily add a date picker component to our Angular app. Here’s an example of how we can do that:
<input type="text" class="form-control" placeholder="yyyy-mm-dd"
name="dp" [(ngModel)]="model" ngbDatepicker
# d="ngbDatepicker">
<button (click)="d.toggle()" class="btn btn-outline-secondary calendar-btn">Select a date</button>
In this code, we have a text input field and a button that triggers the date picker when clicked. The [(ngModel)] directive is used to bind the selected date to a variable named model. We can also customize the format of the date by changing the placeholder attribute.
Modal
Modals are used to display content on top of the current page, usually to grab the user’s attention or ask for confirmation. In Bootstrap, modals are created using the ngb-modalcomponent. Here’s an example of how we can use it in our Angular app:
<button class="btn btn-primary" (click)="open(content)">Launch demo modal</button>
<ng-template
# content let-modal>
<div class="modal-header">
<h4 class="modal-title">Modal title</h4>
<button type="button" class="close" aria-label="Close" (click)="modal.dismiss('Cross click')">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
This is the modal body.
</div>
<div class="modal-footer">
<button type="button" class="btn btn-outline-dark" (click)="modal.close('Save click')">Save</button>
</div>
</ng-template>
In this code, we have a button that triggers the modal when clicked. The `content` template reference variable is used to define the content of the modal. We can also add a header and footer to our modal by using the appropriate Bootstrap classes.
Table
Tables are commonly used to display data in a structured format. ng-bootstrap provides us with a ngb-table component that allows us to create tables in our Angular app easily. Here’s an example of how we can do that:
<table ngbTable
# table [data]="data" [columns]="columns">
<tr *ngFor="let row of table.data">
<td *ngFor="let cell of row.cells">{{ cell }}</td>
</tr>
</table>
In this code, we have defined the data and columns for our table in the component file. Then, we use the ngbTable directive to bind the data and columns to our table. We can also customize the appearance of our table by adding Bootstrap classes to the table element.
Text Inputs and Dropdowns
Bootstrap provides us with various styles for text inputs and dropdowns, making it easier to create forms in our Angular app. Here’s an example of how we can use these components:
<form>
<div class="form-group">
<label for="exampleFormControlInput1">Email address</label>
<input type="email" class="form-control" id="exampleFormControlInput1" placeholder="[email protected]">
</div>
<div class="form-group">
<label for="exampleFormControlSelect1">Example select</label>
<select class="form-control" id="exampleFormControlSelect1">
<option>1</option>
<option>2</option>
<option>3</option>
<option>4</option>
<option>5</option>
</select>
</div>
<div class="form-group">
<label for="exampleFormControlTextarea1">Example textarea</label>
<textarea class="form-control" id="exampleFormControlTextarea1" rows="3"></textarea>
</div>
</form>
In this code, we have a form with three different input fields – email, select, and textarea. We can also add validation to these fields by using Bootstrap’s built-in validation classes.
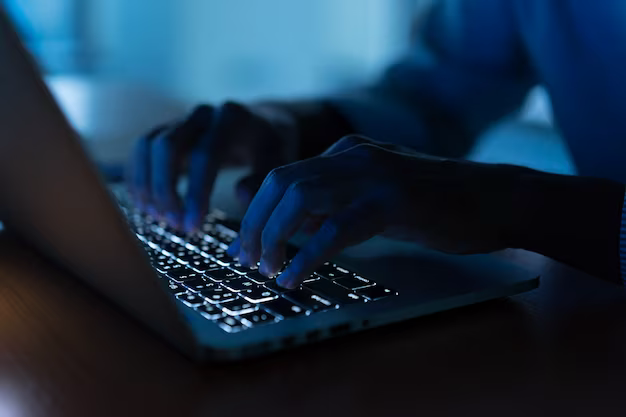
Bootstrap Theming
One of the best things about Bootstrap is its ability to be easily customized and themed. With ng-bootstrap, we can also apply custom themes to our Angular app. To do so, we need to import the Bootstrap SCSS files into our project.
First, we need to install the bootstrap package using npm:
npm install bootstrap
Then, we can import the necessary SCSS files in our styles.scss file:
@import "~bootstrap/scss/bootstrap";
@import "~@ng-bootstrap/ng-bootstrap/index";
Now, we can use Bootstrap’s variables and mixins to customize our app’s theme. For example, we can change the primary color of our app by overriding the $primary variable in the variables.scss file.
Angular Child Routes
In addition to integrating Bootstrap elements, Angular also offers the functionality of child routes. This feature allows for the modularization of routes within an Angular application, enhancing its scalability and maintainability. Child routes can be utilized to encapsulate specific features or sections of an application, enabling a more granular approach to routing and navigation.
By incorporating child routes into our Angular application alongside Bootstrap integration, we can construct highly organized and responsive web applications that adhere to modern design standards. This amalgamation empowers developers to create complex yet intuitive user experiences, ensuring the seamless navigation and interaction within the application.
Conclusion
In this article, we have learned how to install and use Bootstrap in Angular using the ng-bootstrap library. We have also explored some of the most commonly used components and seen how we can integrate them into our Angular app. Additionally, we have learned how to apply custom themes to our app using Bootstrap’s SCSS files.