Angular, as a powerful front-end framework, offers a robust routing system that allows developers to create single-page applications (SPAs) efficiently. In this article, we will delve into the concept of child routes, also known as nested routes, and explore how they can elevate your Angular application’s routing structure. We’ll cover everything from setting up child routes to defining and integrating them within your application. By the end of this comprehensive guide, you will have a solid understanding of how to effectively implement child routes in Angular 12.
Before You Start
Before delving into the intricacies of child routes, it’s essential to ensure that you have the necessary tools and prerequisites in place to seamlessly integrate child routes into your Angular application. Here are the key requirements to get started:
Prerequisites
- An integrated development environment (IDE) such as VS Code;
- Node Package Manager version 6.7 or above;
- The latest version of Angular (version 12): ng version.
Ensure that you are using Angular version 12 and update to this version if you are not already. Additionally, having a working knowledge of the Angular Router at a beginner level would be beneficial. If needed, you can review our previous article on Angular routing to refresh your understanding.
What Are Nesting Routes?
Understanding the concept of nesting routes is crucial for comprehending the power and flexibility it brings to Angular applications. Angular, being a component-based framework, organizes applications into components, forming a hierarchical tree structure. This means that sub-components stem from other components, creating a clear and organized architecture within the application.
For instance, in an Angular app, various components are typically found inside the main app component. Similarly, the Angular Router enables the creation of routes that are nested inside already-defined routes, allowing for a structured and organized navigation flow within the application.
Benefits of Nesting Routes
Nesting routes provides a component-like structure for the routes in your application, offering several advantages:
- Modularity: Child routes enable the encapsulation of specific features or sections of the application, promoting modularity and maintainability;
- Enhanced User Experience: By structuring routes hierarchically, you can control access to certain views, ensuring that users can only navigate to specific views when in a particular context;
- Code Reusability: Child routes facilitate the reuse of components and logic across different sections of the application, leading to cleaner and more maintainable code.
What We Will Be Building?
In this section, we will outline the structure of the application we aim to build to demonstrate the implementation of child routes. Our sample application will consist of a parent component with nested child components, showcasing a practical use case for employing child routes within an Angular application.
Application Structure Overview
Component | Description |
---|---|
ParentComponent | Main parent component serving as the entry point for the application. |
ChildComponent | Nested child component contained within the ParentComponent. |
The application will feature a clear hierarchy, with the ParentComponent acting as the primary container for the ChildComponent, illustrating the seamless integration of child routes within the application.
Setting Up
To begin implementing child routes in Angular, we need to follow a series of steps to define and integrate these routes within our application. The process involves defining parent routes, registering them, setting up templates, generating child components, and adding child routes along with the presentation code.
Defining Parent Routes
The first step is to define the parent routes within the Angular application. These routes will serve as the foundation upon which the child routes will be nested.
Route Definition Process
- Open the app-routing.module.ts file in your Angular project;
- Define the parent route using the RouterModule.forRoot() method, specifying the path and component for the parent route.
const routes: Routes = [
{ path: 'parent', component: ParentComponent }
];
- Save the changes and proceed to the next step.
Registering Them
Once the parent routes have been defined, the next step is to register these routes within the application to ensure their accessibility and functionality.
Route Registration Process
- Open the app.module.ts file in your Angular project;
- Import the RouterModule and Routes from @angular/router;
- Register the defined routes using the RouterModule.forRoot() method within the importsarray of the @NgModule decorator.
import { RouterModule, Routes } from '@angular/router';
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppModule { }
By following these steps, the parent routes are now successfully defined and registered within the Angular application, laying the groundwork for the integration of child routes.
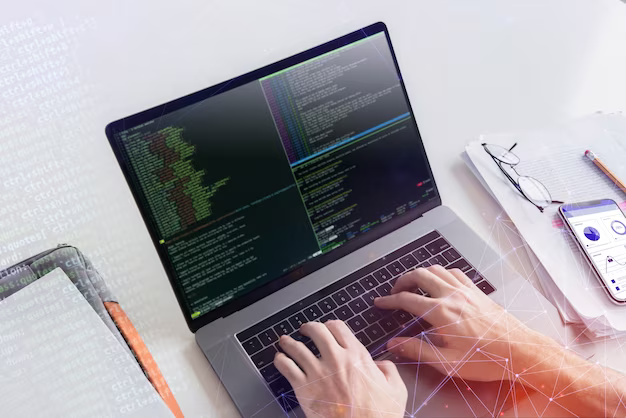
Template Setup
With the parent routes in place, the next step involves setting up the templates for the parent and child components, establishing the visual structure of the application.
Template Configuration
- Create the template for the ParentComponent, incorporating the necessary HTML and styling to define its layout and appearance;
- Similarly, create the template for the ChildComponent, ensuring that it complements the design and functionality of the ParentComponent.
By configuring the templates for both the parent and child components, you establish the visual foundation for the application, preparing it for the integration of child routes.
Testing It Out
After setting up the templates and registering the parent routes, it’s crucial to test the application to ensure that the parent route is functioning as expected before proceeding to integrate child routes.
Testing Procedure
- Run the Angular application using the ng serve command;
- Navigate to the specified path for the parent route in a web browser to verify that the parent component is rendered correctly.
By testing the parent route, you can confirm that the initial setup has been executed accurately, providing a solid base for integrating child routes seamlessly.
Generate Child Components
The next phase involves generating the child components that will be nested within the parent component, forming the core structure of the child routes.
Component Generation Process
- Use the Angular CLI to generate the child components by running the following command in the terminal:
ng generate component ChildComponent
- Once generated, the child component files will be created, including the necessary TypeScript, HTML, and CSS files.
By generating the child components, you lay the groundwork for integrating child routes within the application, setting the stage for a hierarchical navigation structure.
Adding Child Routes
After generating the child components, the subsequent step is to add child routes to the existing parent route, enabling the nesting of child components within the parent component.
Child Route Integration Process
- Open the app-routing.module.ts file in your Angular project;
- Define the child routes within the parent route using the children property, specifying the path and component for each child route.
const routes: Routes = [
{
path: 'parent',
component: ParentComponent,
children: [
{ path: 'child', component: ChildComponent }
]
}
];
- Save the changes and proceed to the final step of adding the presentation code.
By adding child routes to the parent route, you establish a nested structure within the application, allowing for a seamless transition between the parent and child components.
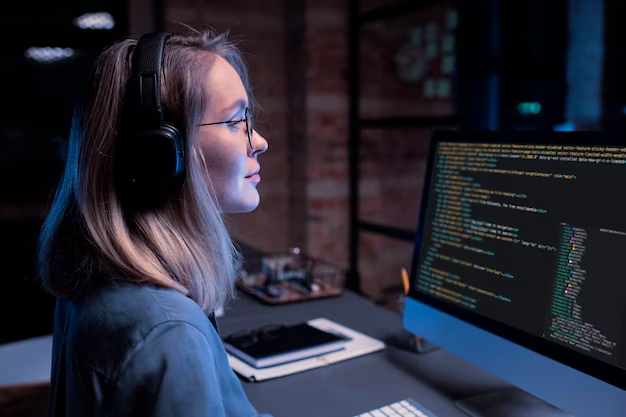
Adding the Presentation Code
The final step involves adding the necessary presentation code to render the child components within the parent component, completing the integration of child routes within the application.
Presentation Code Integration
- Open the template file for the ParentComponent (parent.component.html);
- Use the <router-outlet> directive to specify the location where the child components will be rendered within the parent component’s template.
<div>
<!-- Other content within the parent component -->
<router-outlet></router-outlet>
</div>
By integrating the presentation code, you enable the seamless rendering of child components within the parent component, finalizing the implementation of child routes within the Angular application.
Angular Get Form Values
Retrieving form values in Angular is a common task when working with user input. Angular offers two main mechanisms for accessing form values: template-driven forms and reactive forms.
Template-Driven Forms
In template-driven forms, form values can be accessed using directives and template reference variables.
Reactive Forms
Reactive forms manage form values programmatically using form controls.
By integrating form value retrieval mechanisms with child routes, you can create dynamic and interactive Angular applications that respond to user input while maintaining a structured routing system.
Wrapping Up
With the completion of the aforementioned steps, you have successfully set up and integrated child routes within your Angular application, creating a hierarchical navigation structure that enhances the modularity and organization of your application’s routing system. By leveraging child routes, you have unlocked the potential to build more complex and feature-rich SPAs while maintaining a structured and maintainable codebase.